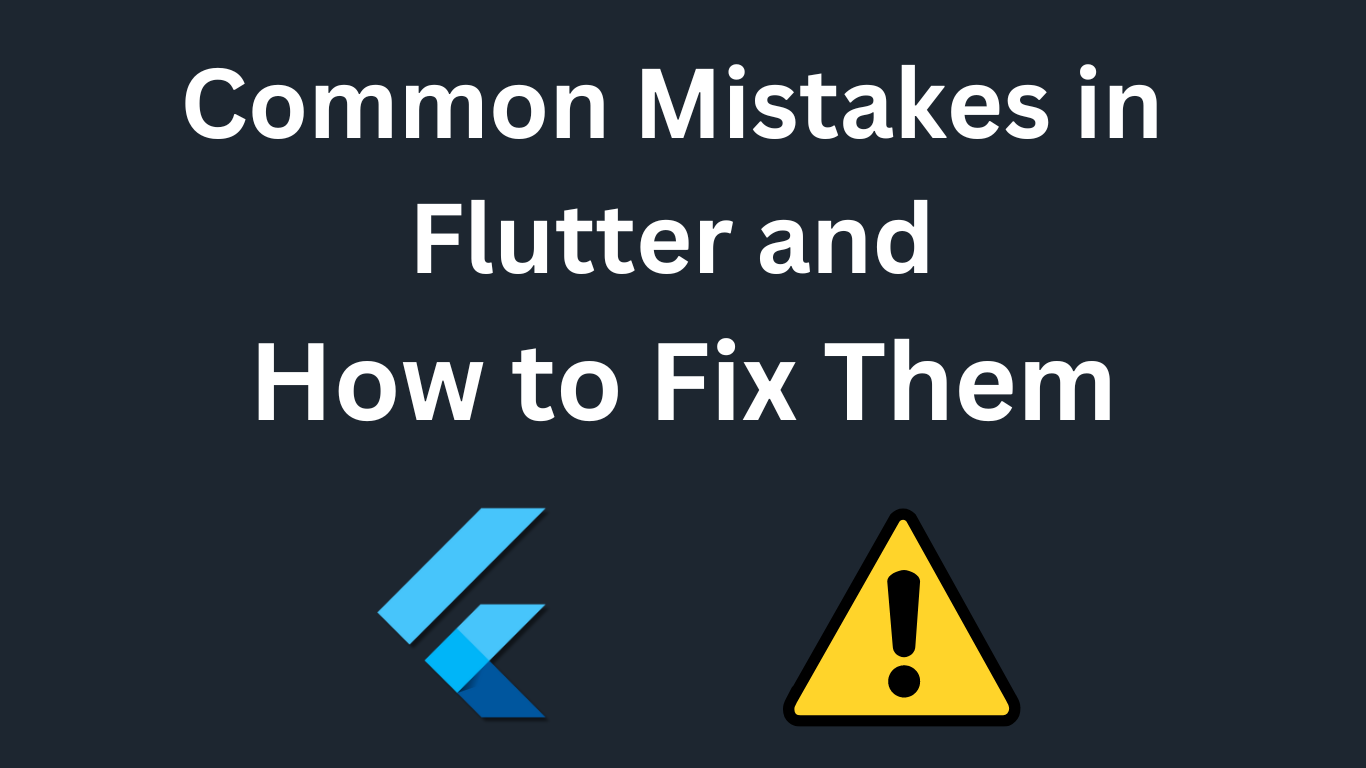
Common Mistakes in Flutter and How to Fix Them – 2024
Flutter, with its hot reload and rich UI capabilities, has become a darling of the app development world. However, even seasoned developers can stumble upon common pitfalls. This guide explores these roadblocks and offers solutions to keep your Flutter app development smooth sailing.
Mismanaging State with setState() overuse:
The Problem: Beginners often rely heavily on setState() for every minor UI update. This triggers unnecessary rebuilds of the widget tree, impacting performance and code clarity.
The Fix: Embrace state management solutions like Provider or Riverpod. These separate business logic from the UI, minimizing unnecessary updates.
The Null Reference Trap:
The Problem: Null references, where a variable lacks a value, can cause crashes.
The Fix: Utilize null checks and conditional statements. For instance, use if (variable != null) before accessing the variable’s properties. Alternatively, consider the null-safety features introduced in Flutter 2, which can help prevent null-related errors at compile time.
Unbounded Layouts and the Overflowing River:
The Problem: Unconstrained widgets can lead to layout errors like “RenderFlex overflowed.” This often occurs when a vertically expanding element (like a ListView) resides within a Column with no height restriction.
The Fix: Employ constraints like SizedBox or Flexible to define the height of widgets within a Column. Alternatively, wrap the overflowing widget with an Expanded widget.
String vs. Integer Mismatch:
The Problem: Attempting operations meant for integers (like adding) on strings leads to errors like “String is not a subtype of int.”
The Fix: Ensure you’re dealing with the correct data type. Use int.parse() to convert strings to integers before performing numerical operations.
BuildContext Blunders:
The Problem: BuildContext, which provides information about the widget tree’s location, can be misused. Forgetting its context-specific nature can cause issues.
The Fix: Grasp the concept of the widget tree. Recognize that changes made through BuildContext only affect the subtree rooted at that specific context.
Forgetting Asynchronous Glory:
The Problem: Flutter is asynchronous at its core. Failing to handle asynchronous operations properly can lead to unexpected behavior.
The Fix: Embrace async/await or FutureBuilder widgets to manage asynchronous data fetching and UI updates gracefully.
Neglecting Error Handling:
The Problem: Unhandled errors during development can snowball into major issues later.
The Fix: Implement proper error handling using try-catch blocks or error listeners. Provide informative error messages to aid debugging.
Overlooking Accessibility:
The Problem: Inaccessible apps alienate a significant portion of users.
The Fix: Integrate accessibility features from the outset. Utilize widgets like Semantics and implement proper focus management to create an inclusive user experience.
Code Duplication Demons:
The Problem: Duplicating code makes maintenance cumbersome and error-prone.
The Fix: Extract reusable components and functions to promote code maintainability. Explore Flutter’s rich widget library for pre-built components whenever possible.
The Deep Nest Woes:
The Problem: Excessively nested widget hierarchies can hinder performance and readability.
The Fix: Strive for a balanced widget tree structure. Consider using Inherited Widget or Provider for state management to reduce nesting complexity.
Bonus Tip: Leverage the Community!
Flutter boasts a vibrant developer community. Don’t hesitate to seek help on forums or explore online resources for solutions and best practices.
How To Install Flutter SDK On Windows 10/11 – Step By Step Guide
By understanding and avoiding these common pitfalls, you can ensure your Flutter development journey is efficient, enjoyable, and leads to exceptional apps. Remember, continuous learning and keeping up-to-date with the latest Flutter practices are key to mastering this powerful framework.