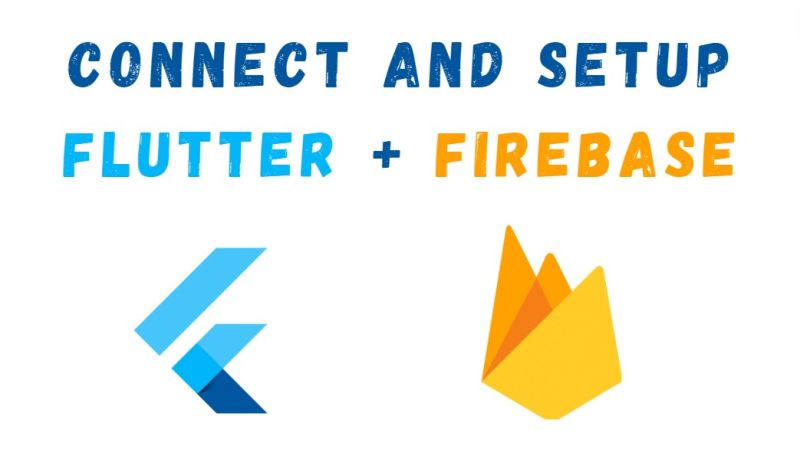
How To Connect Firebase With Flutter Using Flutterfire
Firebase by Google empowers developers with a robust suite of tools for building mobile and web applications. Integrating Firebase with your Flutter app unlocks a treasure trove of features, including user authentication, database management, cloud storage, analytics, and more. This article equips you with a step-by-step guide on connecting Firebase to your Flutter project using FlutterFire, the official set of plugins designed specifically for this purpose.
Prerequisites:
- A functional Flutter project set up on your development machine. how to install flutter
- A Firebase project created and configured. You can create a free Firebase project at https://console.firebase.google.com/.
- Basic understanding of Flutter development concepts.
- Node.js and the Firebase CLI installed on your system. Installation guides can be found here:
- Node.js: https://nodejs.org/en
- Firebase CLI: https://firebase.google.com/docs/cli
Configure Firebase Project:
Log in to your Firebase console and navigate to your project.
In the project overview, click on “Add app” and choose “Web” or “Android” (depending on your target platform) to register your Flutter app with Firebase.
Download the generated configuration file, google-services.json. This file holds your Firebase project configuration details. Rename it to google_services.json (lowercase ‘g’) for consistency.
Install FlutterFire Packages:
Open your terminal and navigate to your Flutter project directory.
Run the following command to install the core Firebase package:
flutter pub add firebase_core
Additionally, install specific FlutterFire packages for the Firebase features you intend to utilize. For instance, to use Firebase Authentication:
flutter pub add firebase_auth
Refer to the official documentation for a complete list of FlutterFire packages: https://firebase.google.com/docs/flutter/setup
Add Firebase Configuration:
Create a new Dart file (e.g., firebase_options.dart) in your project’s root directory.
Utilize the flutterfire configure command to generate the Firebase configuration code snippet. Follow the on-screen prompts to select your Firebase project and target platform. This command will automatically populate the firebase_options.dart file with your project’s configuration details.
Initialize Firebase (Crucial Step):
In your main.dart file, import the necessary packages and Initialize Firebase:
import 'package:firebase_core/firebase_core.dart';
import 'package:firebase_options/firebase_options.dart';
Future main() async {
WidgetsFlutterBinding.ensureInitialized();
await Firebase.initializeApp(
options: DefaultFirebaseOptions.currentPlatform,
);
runApp(MyApp());
}
Explanation:
WidgetsFlutterBinding.ensureInitialized()
ensures that widgets can be rendered before proceeding.await Firebase.initializeApp
is an asynchronous function that waits for Firebase to be fully initialized before continuing with app execution.DefaultFirebaseOptions.currentPlatform
automatically retrieves the appropriate configuration for your target platform (Android or web).
Using Firebase Services:
Once Firebase is initialized, you can access various Firebase features through their corresponding FlutterFire plugins. The official documentation provides detailed information on specific functionalities and usage patterns for each plugin: https://firebase.google.com/docs/flutter/setup
Additional Considerations:
- Platform-Specific Configuration: While the core steps remain similar, there might be slight variations in configuration for Android and web platforms. Refer to the official documentation for specific platform considerations.
- Security: Always prioritize the security of your google_services.json file. Avoid committing it to your version control system (e.g., Git). Consider using environment variables to store sensitive information.
Watch Video on YouTube: Firebase Integration in Flutter with FlutterFire CLI | Step-by-Step Guide
Conclusion:
By following these comprehensive steps, you’ve successfully integrated Firebase with your Flutter app using FlutterFire. This empowers you to leverage a vast array of Firebase features, significantly enhancing your app’s functionality, user experience, and scalability. Explore the extensive set of Firebase features and their corresponding FlutterFire plugins to unlock the full potential of your Flutter development journey.