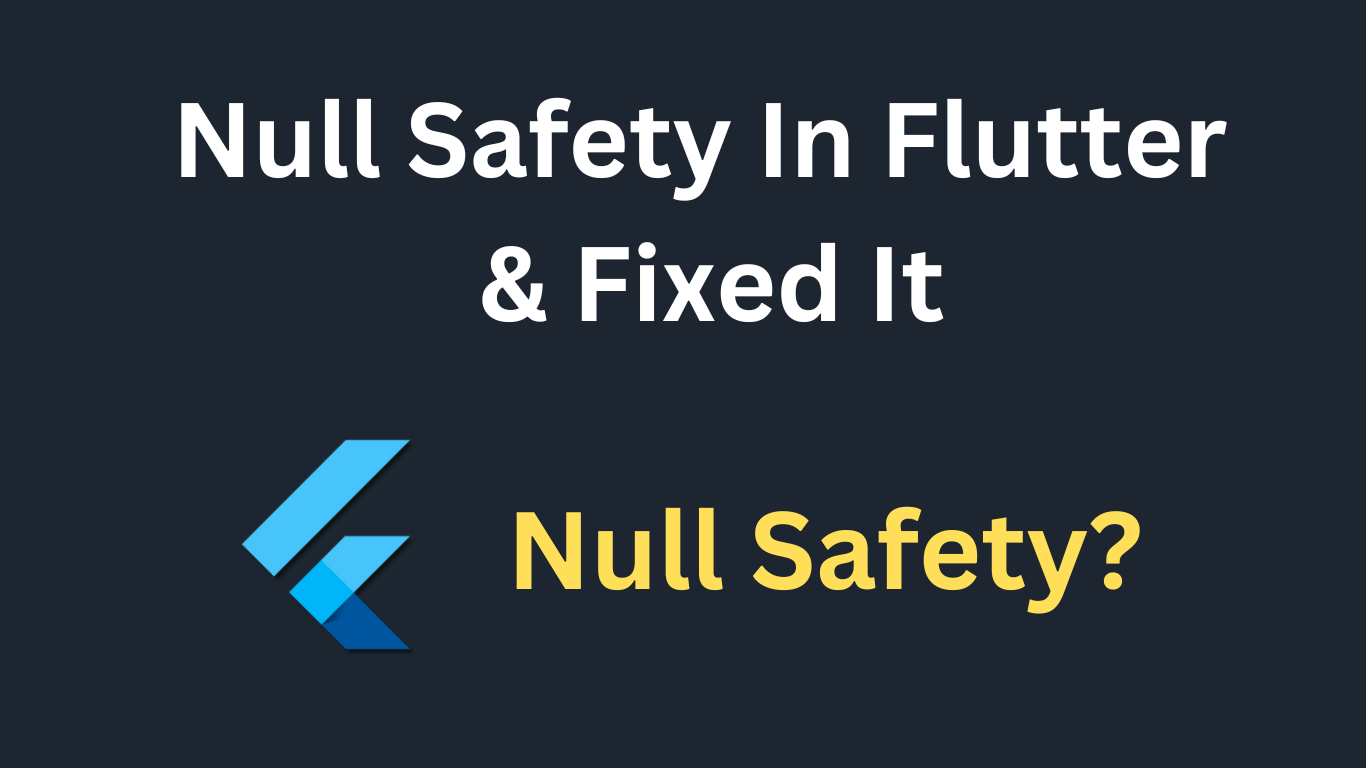
Understanding Null Safety in Flutter and Preventing Errors 2024
For developers building beautiful and functional user interfaces with Flutter, ensuring code stability and preventing crashes is paramount. One of the most common pitfalls developers encounter in Dart, the programming language behind Flutter, is the dreaded null reference error. This occurs when you try to access a property or method on a variable that holds a null value.
Null safety in Dart is a game-changer, introduced to eliminate these errors and make your Flutter applications more robust. Let’s delve into what null safety is, how it works, and the benefits it brings to your development process.
What is Null Safety?
Imagine a variable as a container that can hold a specific type of data, like a name (string), age (integer), or a list of items. Before null safety, these variables could potentially hold either a valid value or be empty, represented by the value null. This inherent uncertainty could lead to errors at runtime, often causing crashes when you attempt to use a null value as if it held actual data.
Null safety introduces a concept of nullable and non-nullable types. A non-nullable type, declared without a question mark (e.g., int age), guarantees that the variable will always hold a valid integer value. Assigning null to a non-nullable variable will result in a compile-time error, alerting you to the potential issue before your app even runs.
On the other hand, a nullable type (e.g., int? maybeAge) indicates that the variable can hold either an integer value or null. This flexibility is useful in situations where a value might not always be available, but care needs to be taken when working with nullable variables.
Benefits of Null Safety
By enforcing null safety, Dart helps you write more predictable and stable Flutter applications. Here are some key advantages:
- Reduced Runtime Errors: Null reference errors become a thing of the past. The compiler identifies potential null issues during the development phase, preventing crashes and unexpected behavior in your app.
- Improved Code Readability: Explicitly declaring nullable and non-nullable types makes your code clearer and easier to understand for yourself and other developers. It becomes self-documenting, as the types communicate how variables are expected to be used.
- Enhanced Maintainability: With null safety, you can refactor and modify your codebase with greater confidence. The compiler acts as a safety net, ensuring that changes don’t inadvertently introduce null-related errors.
- Static Analysis: Powerful static analysis tools can leverage null safety information to provide better code completion, type checking, and refactoring suggestions within your IDE. This streamlines development and helps you write cleaner code.
How to Fix Null Safety Issues
If you’re working on a pre-existing Flutter project that doesn’t yet leverage null safety, you’ll need to migrate your codebase to adopt this feature. Here’s a general approach:
- Enable Null Safety: Update your pubspec.yaml file to set the minimum Dart SDK version that supports null safety (typically version 2.12 or higher).
- Analyze Dependencies: Use the dart pub outdated –mode=null-safety command to identify any dependencies that haven’t yet migrated to null safety.
- Update Dependencies: Look for null-safe versions of your dependencies and update them in your pubspec.yaml file.
- Gradual Migration: Address null safety issues in your code incrementally. Start by focusing on core functionalities and gradually migrate other parts of your codebase.
While migrating to null safety might require some initial effort, the long-term benefits are significant. You’ll end up with a more robust, maintainable, and less error-prone Flutter application.
Beyond the Basics: Advanced Null Safety Concepts
As you delve deeper into null safety, you’ll encounter some advanced concepts:
- Null Assertions: In specific scenarios, you might be certain that a nullable variable holds a value. You can use the null assertion operator (!) to tell the compiler to proceed as if the value is non-null. However, use this with caution, as a mistaken assertion can lead to runtime errors if your assumption is wrong.
- Null-aware Operators: Dart provides null-aware operators (?. and ??) that help you safely access properties or call methods on nullable variables. These operators check for null before proceeding, preventing errors.
Exploring these concepts and best practices will further enhance your understanding and ability to leverage null safety effectively in your Flutter development journey.
Common Mistakes In Flutter And How To Fix Them – 2024
Should You Learn Flutter In 2024? Exploring The Pros And Cons
How To Install Flutter SDK On Windows 10/11 – Step By Step Guide
By embracing null safety, you’re setting yourself up for a smoother, more efficient, and enjoyable Flutter development experience. With the compiler acting as your guardian against null-related pitfalls, you can focus on building beautiful and functional user interfaces with confidence.